Fix: Non Static Method Cannot be Referenced from a Static Context
Many programmers face the error message ‘Non static method cannot be referenced from a static context’ when they are coding. This error message isn’t specific and can occur in any IDE if the conditions for the error are true.
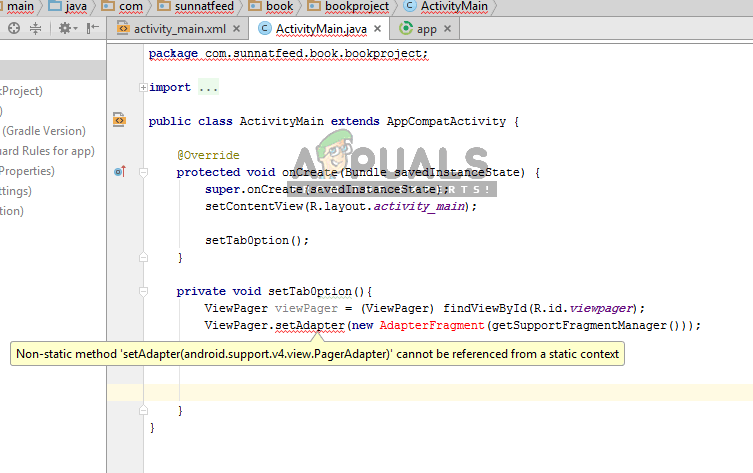
This is a very common mistake for beginners where the programmer tries to use a class ‘statically’ without making an instance of the class. There are several conditions which must be met when you are using a class which is static. In this article, we will go through several different cases and guide you on how to use a static class.
What is a Static Method?
Adding the keyword ‘static’ to any method makes the method known as a static method. A static method belongs to the class rather than belonging to an object (which is the norm). A static method can be easily invoked without the condition of creating an instance of a class.
There are several different uses of Static methods for example, using it, you can change a static data member and its value. However, there are still some limitations when using a Static method. For example, if you want to access non-static fields of your class, you must use a non-static method. So to sum up, Static methods are used very sparely but they do have their benefits.
Here is a short example of how a static method can be made to change the property of all objects.
class Students{ int roll_no; String name; static String college = "InformationTech"; static void change(){ college = “Management"; } Students (int number, String name_self){ roll_no = number; name = name_self; } void display (){System.out.println(rollno+" "+name+" "+college);} public static void main(String args[]){ Students.change(); Students stu_1 = new Students (100,"Mexican"); Students stu_2 = new Students (202,"American"); Students stu_3 = new Students (309,"British"); stu_1.display(); stu_2.display(); stu_3.display(); } }
The output of the program will be:
100 Mexican Management 202 American Management 309 British Management
What is the Difference between a class and instance of a class?
Think you are walking on the street and you see a car. Now you immediately know that this is a car even if you don’t know what is its model or type. This is because you know that this belongs to the class of ‘cars’ which you already know of. Think of class here as a template or an idea.
Now as you move closer, you see the model and make of the car. Here you are recognizing the ‘instance’ of the class ‘car’. Here all the properties will be present in detail; the wheels, the horsepower, the rims etc.
An example of properties can be that the class ‘car’ states that all cars should have wheels. The car which you are seeing (an instance of the car class) has alloy rims.
In object-oriented programming, you define the class yourself and inside the class, you define a field of the type ‘color’. Whenever the class is instantiated, memory is automatically reserved for the color at the backend and later on, you can give this a specific value (for example red). Since attributes like these are specific, they are non-static.
In contrast, static methods and fields are shared with all the instances. These are made for value or items which are specific to the class and not the instance itself. For methods, there can be global methods (for example, stringtoInt converter) and for fields, they are usually constants according to your code (for example, the car type can be static if you are only manufacturing normal cars).
Now, we will look at all the different cases where your coding can go wrong and see the workarounds to fix them.
Issue 1: You are calling something which doesn’t exist
We came across some cases where users were using both static and non-static methods with each other. When we do this, you should be careful of what method is calling what (in terms of static or not). Take a look at the following code:
private java.util.List<String> someMethod(){ /* Some Code */ return someList; } public static void main(String[] strArgs){ // The following statement causes the error. You know why.. java.util.List<String> someList = someMethod(); }
Here, the static method is calling someMethod. In object-oriented programming, we encapsulate the data together with the data which we want to operate on. Here, without an object, there is no instance data and while the instance methods exist as part of the class definition, there should always be an object instance to provide data to them.
So to sum up, you cannot call something which doesn’t exist. Since you might not have created an object, the non-static method doesn’t exist yet. However, on the other hand, a static method will always exist (because of definition).
Issue 2: Methods aren’t made Static
If you are invoking methods from your Static main method without creating an instance of the methods, you will get an error message. Here, the same principle applies; you cannot access something that doesn’t exist.
public class BookStoreApp2 { //constants for options Scanner input = new Scanner (System.in); public static void main(String[] args) { BookStoreItem[] item;//declaring array item = new BookStoreItem[10];//initializing array int itemType = -1; printMenu(); getUserChoice(); for (int i = 0; i < item.length; i++){ }//end of switch statement }//end of for loop for (int i = 0; i < item.length; i++) { }//end of for }//end of main method
Here in this code, you need to convert both the methods printMenu() and getUserChoice() into static methods.
Hence if you want to get around a situation like this, you can use a constructor instead. For example, you can take the contents of your main() method and place them inside a constructor.
public BookStoreApp2() { // Put contents of main method here} After doing this, do the following inside your main() method: public void main( String[] args ) { new BookStoreApp2(); }
If these tips don’t work on your or your code is different, you should keep in mind the basic principles of Static classes and methods and recheck your code to make sure that the basic principle is not being violated.